Authorization Flow
Basics of Authorization
Overview
We use OAuth2 to allow your application (CRM) to access our sites on the user's behalf. This required in order for each individual user to give consent to your 3rd party service.
The authorization server is responsible for issuing authorization codes and token that will be used to authorize the user in the target site. If you're not familiar with the OAuth2 protocol, check out this page for a step by step reference or Aaron Parecki’s “OAuth 2 Simplified” guide for a more comprehensive guide.
Marketplace Example
For the sake of example, we will be referring to Imovirtual as the site where users will authorize your application to access their accounts.
To see currently available sites, visit our page about the supported sites.
Authorization flow
After storing the Client ID and the Client Secret that were provided to you in a safe place, it's time to authorize your application to manage user's adverts on the site.
The following image shows every step that is performed in the OAuth2 process:
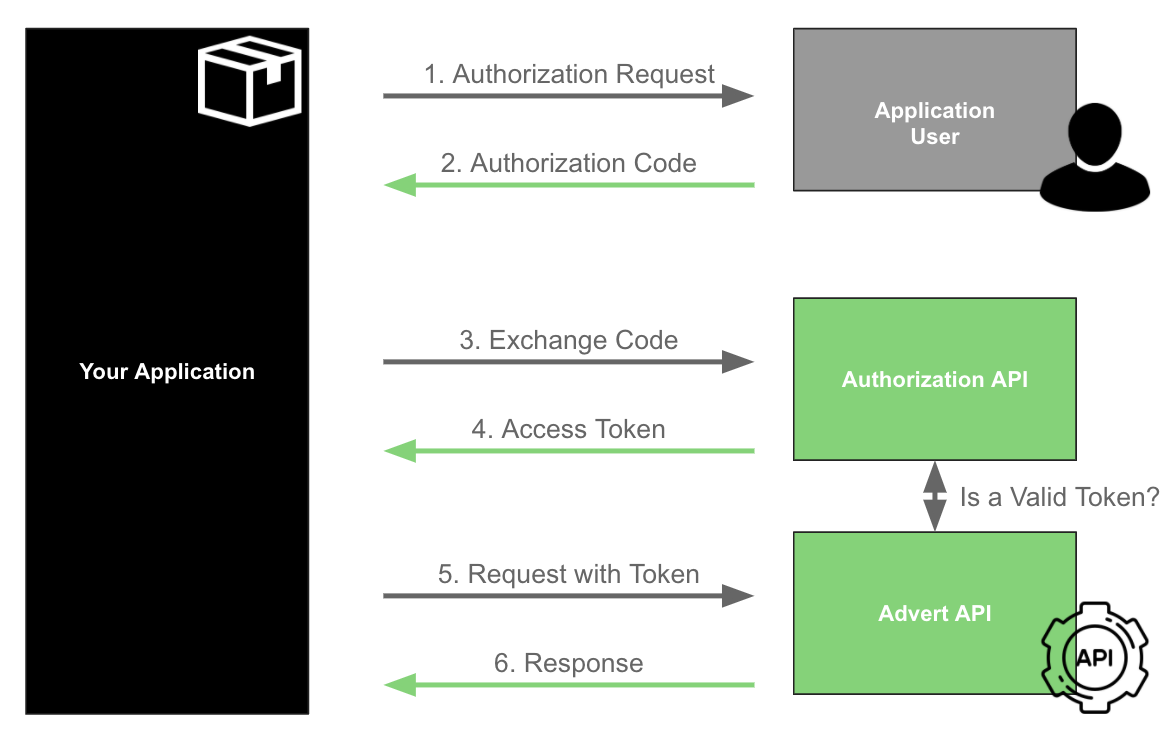
OAuth Diagram
1. User authorizes your app
Each user must be authorized individually
The purpose of OAuth2 is to enable users to give your application consent manage their adverts. Because of this, this process must be done for each user of the site.
The application user accesses the Application (your CRM), which redirects them to the site they want to use (such as Imovirtual.com), so that they can first authenticate on the site and then authorize your application to access data on their account and/or execute actions on their behalf.
You should redirect users to this URL:
https://<OUR RE SITE>/mercury/authorization/?response_type=code&client_id=<YOUR CLIENT ID>&state=<USER ID>
Note that you must include the following parameters in this URL:
Parameter | Description | Value | Notes |
---|---|---|---|
OUR RE SITE | URL of the Portal you want to integrate with. | Check out the supported sites to see which ones are available. | Example: www.imovirtual.com |
response_type | OAuth2 response type. | code is the only supported value. | |
YOUR CLIENT ID | Client ID that was provided to you as part of the application Details. | Application Client ID | Make sure that you configure the correct data for the different environments, in case you have an application for each environment. |
USER ID | Defined as state. It refers to the application user internal identifier from your side, for example. This value will be passed back to you to prevent request tampering (more details below). | Random string | Without this value, you will not be able to automate the process to know the corresponding code for each application user is authorising the application. |
Redirect URI
The OAuth2 protocol also specifies that a redirect URI can be passed as a parameter .You don't need to do this because you already provided this URI when you registered your app (in the field Authorization Callback URI in the Application Manager. However, if you do pass it as a parameter, it will be validated against that one. If the base URIs don't match, your request will fail.
State parameter
The state field should be generated and validated by the Client Application. Its purpose is to help mitigate CSRF attacks. As explained in the OAuth 2.0 RFC, the state must use a non-guessable value and the user-agent's authenticated state (e.g., session cookie, HTML5 local storage).
The user has to provide the correct professional credentials to log in. After logging, the Site (Imovirtual, as an example) redirects users to a secure authorization page. On this page, users must grant your application permission to access the private information in their account. If they refuse to grant permission, then they are redirected back to your app.
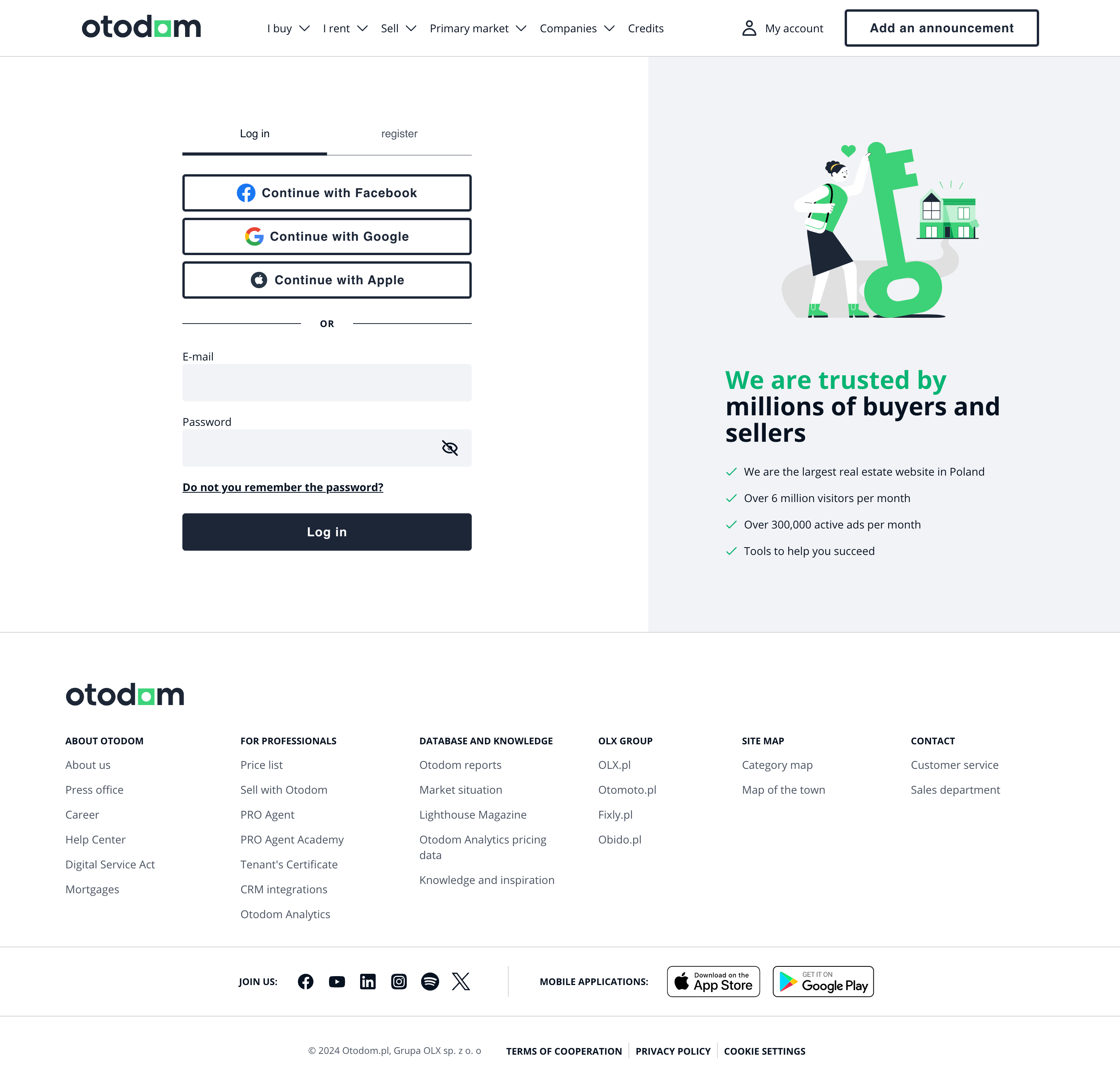
Otodom Login page
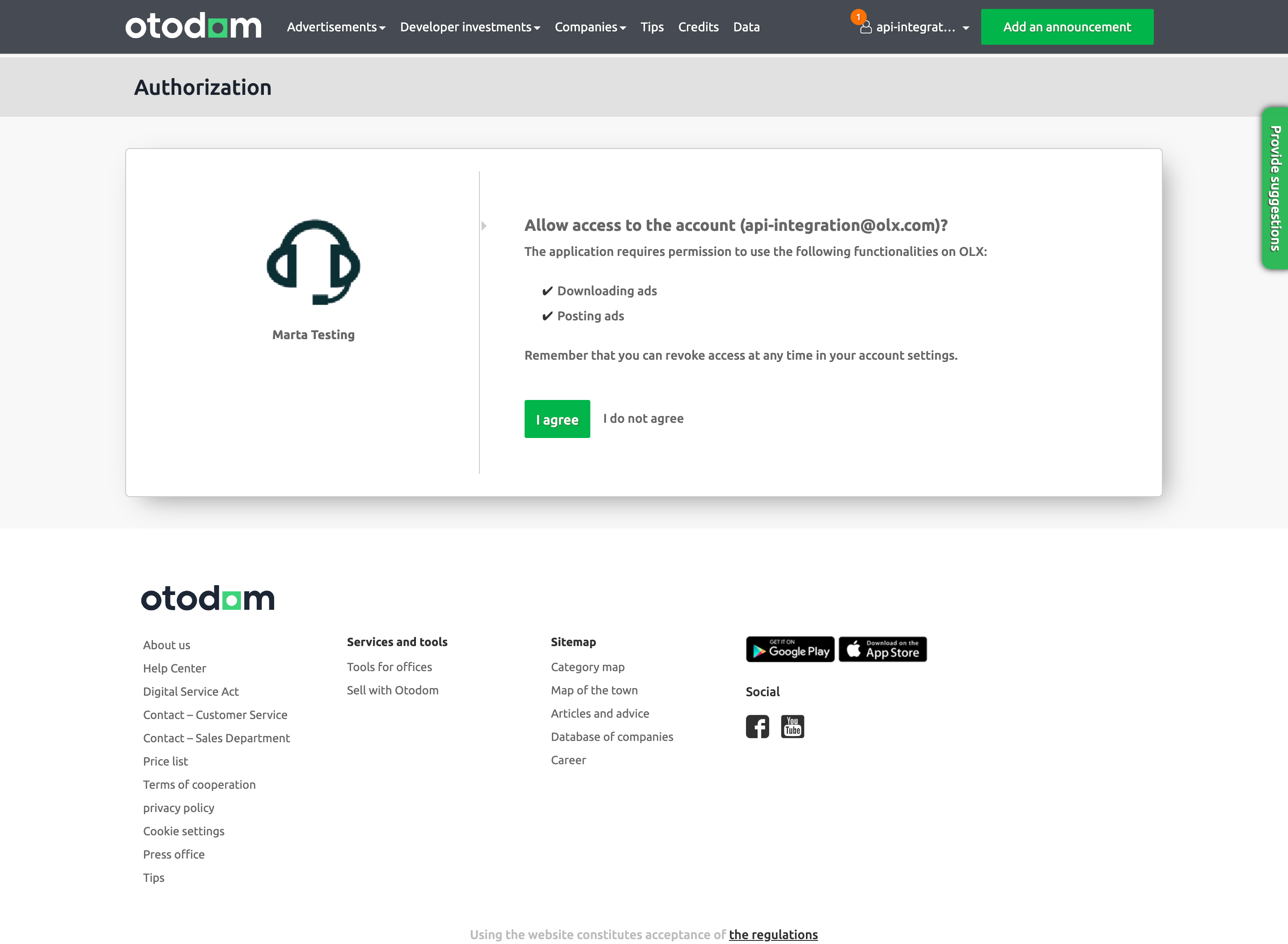
Otodom Authentication page
2. Getting the authorization code
After accepting that your application will authorize their account on the selected site, the user will be redirected back to your application (to the Authorization Callback URI specified in application manager) with an authorization code
and the state
previously sent in the request. For example:
302 Found
Location: https://yourawesomeapp.com/redirect/oauth?code=7faf9c72733ad3472963fdb3cb24b94e2f641a06&state=whateverStateYouHaveSentPreviously
You will know what user is authorizing the application based on the value in state
. You should use thecode
to request their tokens.
3. Exchange code
for an access token
code
for an access tokenThe user is done authorizing, and now you must finish the process! You must now take this code
and exchange it for an access_token
.
Authorization code TTL
The authorization code only lasts 60 seconds. You must request the access token within this timeframe. Otherwise, the user will have to grant your app permissions again.
You can do that by doing a request such as:
curl --location --request POST 'https://api.olxgroup.com/oauth/v1/token' \
--header 'Accept: application/json' \
--header 'Content-Type: application/json' \
--header 'Authorization: Basic <YOUR BASIC CREDENTIALS>' \
--header 'X-API-KEY: <YOUR API KEY>' \
--header 'User-Agent: <CRM NAME>'
--data-raw '{
"grant_type":"authorization_code",
"code":"<CODE>"
}'
The Basic Credentials should be a base64 encoding of your Client ID and Client Secret. You can find this information in your Application Manager. To read more about it, visit this page .
4. Getting an access token for the first time
Here's an example of what you will get from the previous request:
{
"access_token" : "a500491037ee99ed8a68fdd5bd1d756940490a19",
"token_type" : "Bearer",
"refresh_token" : "bd921d60678b32a31cde526c6e5b658b4ea157ba",
"expires_in" : 3600,
"scope" : "read:adverts write:adverts read:leads read:profile_package"
}
Let's make sure we understand what an Access Token Object is and what properties it has.
Property | Description |
---|---|
access_token | A 41 char alpha-numeric string that grants access to resources with a specific scope from a specific user. |
token_type | The token type for which we only support Bearer . You should send it in the Authorization Header like this:-H 'Authorization: Bearer {$access_token}' |
refresh_token | A 41 char alpha-numeric string to be used to refresh the Access Token Object. |
expires_in | An integer expressed in seconds. It's the TTL (time to live) for the access_token property. Our access tokens are valid for 3600 seconds (one hour). |
scope | A concatenated string of scopes. These scopes grant you access to certain areas of the user account and can be configured in your application. |
You're done!
With this token object, you're ready to make authorized requests on behalf of the user.
5. Refreshing the access token
As you can see above, an Access Token Object has several properties. One of those is the expires_in
which is expressed in seconds and describes the TTL of the access_token
property.
When you're making API calls and the access_token
expires, a response like the following one is returned with a 403 Forbidden
HTTP Status Code:
{
"transaction_id": "c793460c-f7bc-11e8-a049-4f7b3b284a42",
"message": "The access token provided has expired"
}
This means you need to refresh your Access Token Object.
To do that, you will need to get your last refresh_token
and perform the following call to the OAuth API:
curl --location --request POST 'https://api.olxgroup.com/oauth/v1/token' \
--header 'Accept: application/json' \
--header 'Content-Type: application/json' \
--header 'Authorization: Basic <YOUR BASIC CREDENTIALS>' \
--header 'X-API-KEY: <YOUR API KEY>' \
--header 'User-Agent: <CRM NAME>'
--data-raw '{
"grant_type":"refresh_token",
"refresh_token":"<YOUR REFRESH TOKEN>"
}'
With that, you'll have a new Access Token Object with a brand new access_token
property and new refresh_token
.
{
"access_token" : "a500491037ee99ed8a68fdd5bd1d756940490a19",
"token_type" : "Bearer",
"refresh_token" : "bd921d60678b32a31cde526c6e5b658b4ea157ba",
"expires_in" : 3600,
"scope" : "read:adverts write:adverts read:leads read:profile_package"
}
Persisting Access Token Objects
We strongly suggest that you persist your Access Token Objects securely so that you can refresh them whenever needed. Also, remember that each Access Token is unique and issued for a specific application user.
Updated 4 months ago
If you followed this guide until now you should have the necessary setup to start publishing ads. Check out the next section to learn more.